創建你的第一個使用 OpenAI ChatGPT API 的程序
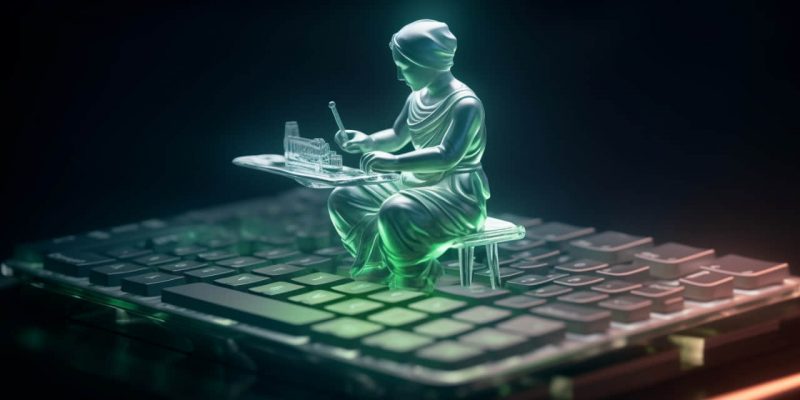
易於使用的 AI 「ChatGPT」 已經以 API 提供。ChatGPT 的創造者 OpenAI 宣布,模型('gpt-3.5-turbo')現在適用於自定義產品和解決方案。而且成本也非常實惠。目前的價格為每 1000 個令牌 0.002 美元。
該模型目前與 Whisper API 一起提供,後者也用於文本到語音解決方案。該 API 目前具備以下功能:
- 創建自定義的對話代理和機器人
- 為你編寫 Python 代碼
- 起草電子郵件或任何你想要的文檔
- 你可以將自然語言界面集成到你當前的產品/應用/服務或軟體中,為你的消費者提供服務
- 語言翻譯服務
- 成為許多科目的導師
- 模擬視頻遊戲角色
正如你所見,機會無限。
如果你計劃嘗試該 API 並開始使用它,這裡有一個簡單的指南,為你提供逐步指導。
OpenAI ChatGPT API: 入門指南
先決條件
確保你擁有一個 OpenAI 賬戶。如果你沒有,訪問此頁面 並創建一個賬戶。你也可以使用你的谷歌或微軟賬號。
創建一個賬戶後,生成一個專屬於你的 API 密鑰。訪問 此頁面 並創建一個新的秘密密鑰。
記錄該密鑰或在安全的地方保存它。基於安全原因,它將不會從 OpenAI 賬戶部分再次可見。而且不要與任何人分享此密鑰。如果你計劃使用企業解決方案,請向你的組織查詢 API 密鑰。由於該密鑰與你的付費 OpenAI 計劃相關,因此請謹慎使用。
設置環境
安裝 Python 和 pip
本指南使用 Python 編程語言來調用 OpenAI API 密鑰。你可以使用 Java 或其他任何語言來調用它。
首先,請確保你在 Linux 或 Windows 中已經安裝了 Python。如果沒有,請按照以下指南安裝 Python。如果你使用現代 Linux 發行版(例如 Ubuntu),Python 應該已經安裝好了。
在安裝 Python 後,確保 pip
在 Linux 發行版中可用。運行以下命令進行安裝。對於 Windows,你應該已經在 Python 安裝的一部分中安裝了它。
Ubuntu、Debian 和其他基於 Debian 的發行版:
sudo apt install python3-pip
Fedora、RHEL、CentOS 等:
sudo dnf install python3-pip
Arch Linux:
sudo pacman -S python-pip
將 OpenAI API 密鑰設置為環境變數
上述步驟中創建的 API 密鑰,你可以直接在程序中使用。但這並不是最佳實踐。
最佳實踐是從文件或你系統的環境變數中調用它。
對於 Windows,請設置一個任何名字的環境變數,例如 API-KEY
。並添加密鑰值。
對於 Linux,請使用超級用戶許可權打開 /etc/environment
文件並添加密鑰。例如:
API-KEY="<你的密鑰>"
對於基於文件的密鑰訪問,請在你的代碼中使用以下語句:
openai.api_key_path = <你的 API 密鑰路徑>
對於直接在代碼中訪問(不建議),你可以在你的代碼中使用以下語句:
openai.api_key = "你的密鑰"
注意:如果驗證失敗,OpenAI API 將拋出以下錯誤。你需要驗證你的密鑰值、路徑和其他參數以進行更正:openai.error.AuthenticationError: No API key provided
。
安裝 OpenAI API
最後一步是安裝 OpenAI 的 Python 庫。打開終端或命令窗口,使用以下命令安裝 OpenAI API。
pip install openai
在此階段,你已經準備好撰寫你的第一個程序了!
編寫助手程序(逐步)
OpenAI API 提供了各種介面模式。例如「聊天補完」、「代碼補完」、「圖像生成」等。在本指南中,我將使用 API 的「聊天補完」功能。使用此功能,我們可以創建一個簡單的對話聊天機器人。
首先,你需要導入 OpenAI 庫。你可以使用以下語句在你的 Python 程序中完成:
import openai
在這個語句之後,你應該確保啟用你的 API 密鑰。你可以使用上面解釋的任何方法來完成。
openai.api_key="your key here"
openai.api_key="your environment variable"
openai.api_key_path = <your path to API key>
OpenAI 聊天 API 的基本功能如下所示。openai.ChatCompletion.create
函數以 JSON 格式接受多個參數。這些參數的形式是 「角色」(role
) 和 「內容」(content
):
openai.ChatCompletion.create(
model = "gpt-3.5-turbo",
messages = [
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "Who won the world series in 2020?"},
{"role": "assistant", "content": "The Los Angeles Dodgers won the World Series in 2020."},
{"role": "user", "content": "Where was it played?"}
]
)
說明:
role
: 有效的值為 system
、user
、assistant
system
: 指示 API 如何行動。基本上,它是 OpenAI 的主提示。user
: 你要問的問題。這是單個或多個會話中的用戶輸入。它可以是多行文本。assistant
: 當你編寫對話時,你需要使用此角色來添加響應。這樣,API 就會記住討論的內容。
注意:在一個單一的消息中,你可以發送多個角色。如上述代碼片段所示的行為、你的問題和歷史記錄。
讓我們定義一個數組來保存 OpenAI 的完整消息。然後向用戶展示提示並接受 system
指令。
messages = []
system_message = input("What type of chatbot you want me to be?")
messages.append({"role":"system","content":system_message})
一旦設置好了,再次提示用戶進行關於對話的進一步提問。你可以使用 Python 的 input
函數(或任何其他文件輸入方法),並為角色 user
設置 content
。
print("Alright! I am ready to be your friendly chatbot" + "n" + "You can now type your messages.")
message = input("")
messages.append({"role":"user","content": message})
現在,你已經準備好了具有基本 JSON 輸入的數組,用於調用「聊天補完」服務的 create
函數。
response=openai.ChatCompletion.create(model="gpt-3.5-turbo",messages=messages)
現在,你可以對其進行適當的格式化,要麼列印響應,要麼解析響應。響應是以 JSON 格式提供的。輸出響應提供了 choices
數組。響應在 message
JSON 對象下提供,其中包括 content
值。
對於此示例,我們可以讀取 choices
數組中的第一個對象並讀取其 content
。
reply = response["choices"][0]["message"]["content"]
print(reply)
最後,它將為你提供來自 API 的輸出。
運行代碼
你可以從你的 喜好的 Python IDE 或直接從命令行運行代碼。
python OpenAIDemo2.py
未格式化的 JSON 輸出
以下是使用未格式化的 JSON 輸出運行上述程序供你參考。正如你所看到的,響應在 choices
數組下具有 content
。
[debugpoint@fedora python]$ python OpenAIDemo2.py
What type of chatbot you want me to be?a friendly friend
Alright! I am ready to be your friendly chatbot
You can now type your messages.
what do you think about kindness?
{
"choices": [
{
"finish_reason": "stop",
"index": 0,
"message": {
"content": "As an AI language model, I don't have personal opinions, but I can tell you that kindness is a very positive and essential trait to have. Kindness is about being considerate and compassionate towards others, which creates positive emotions and reduces negativity. People who are kind towards others are more likely to inspire kindness and compassion in return. It is an important quality that helps to build positive relationships, promote cooperation, and create a more peaceful world.",
"role": "assistant"
}
}
],
"created": <removed>,
"id": "chatcmpl-<removed>",
"model": "gpt-3.5-turbo-0301",
"object": "chat.completion",
"usage": {
"completion_tokens": 91,
"prompt_tokens": 22,
"total_tokens": 113
}
}
格式化的輸出
這是一個適當的對話式輸出。
[debugpoint@fedora python]$ python OpenAIDemo2.py
What type of chatbot you want me to be?a friendly friend
Alright! I am ready to be your friendly chatbot
You can now type your messages.
what do you think about artificial general intelligence?
As an AI language model, I am programmed to be neutral and not have personal opinions. However, artificial general intelligence (AGI) is a fascinating field of study. AGI refers to the development of machines and algorithms that can perform any intellectual task that a human being can. The potential benefits and risks of AGI are still widely debated, with some experts worried about the implications of machines reaching human-like intelligence. However, many believe that AGI has the potential to revolutionize fields such as healthcare, education, and transportation. The key is to ensure that AGI is developed in a responsible and ethical manner.
完整代碼
這是上面演示中使用的完整代碼。
import openai
openai.api_key = "<your key>"
messages = []
system_message = input("What type of chatbot you want me to be?")
messages.append({"role":"system","content":system_message})
print("Alright! I am ready to be your friendly chatbot" + "n" + "You can now type your messages.")
message = input("")
messages.append({"role":"user","content": message})
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=messages
)
reply = response["choices"][0]["message"]["content"]
print(reply)
總結
希望這篇簡單的指南能讓你開始嘗試 OpenAI CharGPT API。你可以將上述步驟擴展到更複雜的會話式聊天機器人。此外,你還可以使用 OpenAI 的其他產品。
請不要錯過我後續的教程,我將會實驗和分享給大家。最後,請不要忘記關注我們,以便及時獲取我們的文章。
如果上述步驟對你有幫助,請在評論框中告訴我。
乾杯!
(題圖:MJ/b206dd48-f698-4800-bccc-19ea11a17ea6)
via: https://www.debugpoint.com/openai-chatgpt-api-python
作者:Arindam 選題:lkxed 譯者:ChatGPT 校對:wxy
本文轉載來自 Linux 中國: https://github.com/Linux-CN/archive