Bash Shell 腳本新手指南(三)
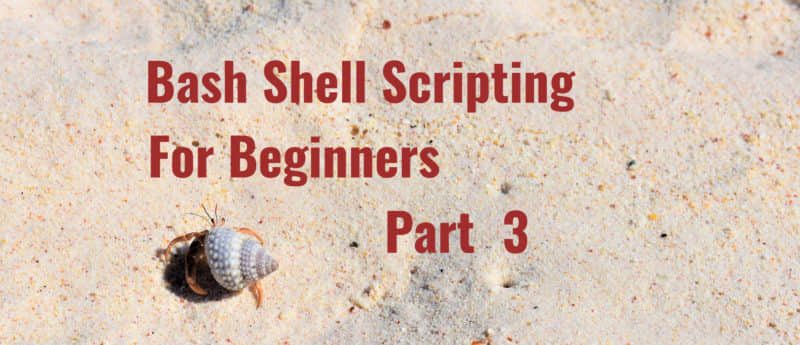
歡迎來到面向初學者的 Bash Shell 腳本知識第三部分。這最後一篇文章將再來學習一些知識點,這些將使你為持續的個人發展做好準備。它將涉及到函數、用 if
/elif
語句進行比較,並以研究 while
循環作為結尾。
函數
讓我們從一個看似困難但其實很簡單的基本概念開始,即函數。把它看作是一種簡單的方法,可以把腳本中被反覆使用的部分放到一個可重複使用的組中。你在本系列第一篇或第二篇文章中所做的任何事情都可以放在一個函數中。因此,讓我們把一個函數放到我們的 learnToScript.sh
文件中。讓我指出幾個關鍵點。你將需要為你的函數起一個名字、一對小括弧,以及用大括弧包圍放在你的函數中的命令。
#!/bin/bash
#A function to return an echo statement.
helloFunc() {
echo "Hello from a function."
}
#invoke the first function helloFunc()
helloFunc
你會看到如下輸出結果:
[zexcon@fedora ~]$ ./learnToScript.sh
Hello from a function.
[zexcon@fedora ~]$
函數是重複使用一組命令的好方法,但如果你能使它們在每次使用時對不同的數據進行操作,它們會更加有用。這就要求你在每次調用函數時提供數據,這稱為參數。
要提供參數,你只需在調用函數時把它們加在函數名稱後面。為了使用你提供的數據,你在函數命令中使用位置來引用它們。它們將被命名為 $1
、$2
、$3
,以此類推,這取決於你的函數將需要多少個參數。
讓我們修改上一個例子來幫助更好地理解這個問題。
#!/bin/bash
#A function to return an echo statement.
helloFunc() {
echo "Hello from a function."
echo $1
echo $2
echo "You gave me $# arguments"
}
#invoke the function helloFunc()
helloFunc "How is the weather?" Fine
輸出如下:
Hello from a function.
How is the weather?
Fine
You gave me 2 arguments
輸出中發生的事情是 helloFunc()
在每一行都做了一個回顯。首先它回顯了一個 Hello from a function
,然後它繼續回顯變數 $1
的值,結果是你傳遞給 helloFunc
的 "How is the weather?"
。然後它將繼續處理變數 $2
,並回顯其值,這是你傳遞的第二個項目:Fine
。該函數將以回顯 You gave me $# arguments
結束。注意,第一個參數是一個用雙引號括起來的單個字元串 "How is the weather?"
。第二個參數 Fine
沒有空格,所以不需要引號。
除了使用 $1
、$2
等之外,你還可以通過使用變數 $#
來確定傳遞給函數的參數數量。這意味著你可以創建一個接受可變參數數量的函數。
關於 bash 函數的更多細節,網上有很多好的參考資料。這裡有一個可以讓你入門的資料。
我希望你能了解到函數如何在你的 bash 腳本中提供巨大的靈活性。
數值比較 []
如果你想進行數字比較,你需要在方括弧 []
中使用以下運算符之一:
-eq
(等於)-ge
(等於或大於)-gt
(大於)-le
(小於或等於)-lt
(小於)-ne
(不相等)
因此,舉例來說,如果你想看 12 是否等於或小於 25,可以像 [ 12 -le 25 ]
這樣。當然,12 和 25 可以是變數。例如,[ $twelve -le $twentyfive ]
。(LCTT 譯註:注意保留方括弧和判斷語句間的空格)
if 和 elif 語句
那麼讓我們用數字比較來介紹 if
語句。Bash 中的 if
語句將以 if
開始, 以 fi
結束。if
語句 以 if
開始,然後是你想做的檢查。在本例中,檢查的內容是變數 numberOne
是否等於 1
。如果 numberOne
等於 1
,將執行 then
語句,否則將執行 else
語句。
#!/bin/bash
numberTwelve=12
if [ $numberTwelve -eq 12 ]
then
echo "numberTwelve is equal to 12"
elif [ $numberTwelve -gt 12 ]
then
echo "numberTwelve variable is greater than 12"
else
echo "neither of the statemens matched"
fi
輸出如下:
[zexcon@fedora ~]$ ./learnToScript.sh
numberTwelve variable is equal to 12
你所看到的是 if
語句的第一行,它在檢查變數的值是否真的等於 12
。如果是的話,語句就會停止,並發出 numberTwelve is equal to 12
的回顯,然後在 fi
之後繼續執行你的腳本。如果變數大於 12
的話,就會執行 elif
語句,並在 fi
之後繼續執行。當你使用 if
或 if
/elif
語句時,它是自上而下工作的。當第一條語句是匹配的時候,它會停止並執行該命令,並在 fi
之後繼續執行。
字元串比較 [[]]
這就是數字比較。那麼字元串的比較呢?使用雙方括弧 [[]]
和以下運算符等於或不等於。(LCTT 譯註:注意保留方括弧和判斷語句間的空格)
=
(相等)!=
(不相等)
請記住,字元串還有一些其他的比較方法,我們這裡不會討論,但可以深入了解一下它們以及它們是如何工作的。
#!/bin/bash
#variable with a string
stringItem="Hello"
#This will match since it is looking for an exact match with $stringItem
if [[ $stringItem = "Hello" ]]
then
echo "The string is an exact match."
else
echo "The strings do not match exactly."
fi
#This will utilize the then statement since it is not looking for a case sensitive match
if [[ $stringItem = "hello" ]]
then
echo "The string does match but is not case sensitive."
else
echo "The string does not match because of the capitalized H."
fi
你將得到以下三行:
[zexcon@fedora ~]$ ./learnToScript.sh
The string is an exact match.
The string does not match because of the capitalized H.
[zexcon@fedora ~]$
while 循環
在結束這個系列之前,讓我們看一下循環。一個關於 while
循環的例子是:「當 1 小於 10 時,在數值上加 1」,你繼續這樣做直到該判斷語句不再為真。下面你將看到變數 number
設置為 1
。在下一行,我們有一個 while
語句,它檢查 number
是否小於或等於 10
。在 do
和 done
之間包含的命令被執行,因為 while
的比較結果為真。所以我們回顯一些文本,並在 number
的值上加 1
。我們繼續執行,直到 while
語句不再為真,它脫離了循環,並回顯 We have completed the while loop since $number is greater than 10.
。
#!/bin/bash
number=1
while [ $number -le 10 ]
do
echo "We checked the current number is $number so we will increment once"
((number=number+1))
done
echo "We have completed the while loop since $number is greater than 10."
while
循環的結果如下:
[zexcon@fedora ~]$ ./learnToScript.sh
We checked the current number is 1 so we will increment once
We checked the current number is 2 so we will increment once
We checked the current number is 3 so we will increment once
We checked the current number is 4 so we will increment once
We checked the current number is 5 so we will increment once
We checked the current number is 6 so we will increment once
We checked the current number is 7 so we will increment once
We checked the current number is 8 so we will increment once
We checked the current number is 9 so we will increment once
We checked the current number is 10 so we will increment once
We have completed the while loop since 11 is greater than 10.
[zexcon@fedora ~]$
正如你所看到的,實現這一目的所需的腳本量要比用 if
語句不斷檢查每個數字少得多。這就是循環的偉大之處,而 while
循環只是眾多方式之一,它以不同的方式來滿足你的個人需要。
總結
下一步是什麼?正如文章所指出的,這是,面向 Bash Shell 腳本初學者的。希望我激發了你對腳本的興趣或終生的熱愛。我建議你去看看其他人的腳本,了解你不知道或不理解的地方。請記住,由於本系列每篇文章都介紹了數學運算、比較字元串、輸出和歸納數據的多種方法,它們也可以用函數、循環或許多其他方法來完成。如果你練習所討論的基礎知識,你將會很開心地把它們與你還要學習的所有其他知識結合起來。試試吧,讓我們在 Fedora Linux 世界裡見。
via: https://fedoramagazine.org/bash-shell-scripting-for-beginners-part-3
作者:Matthew Darnell 選題:lujun9972 譯者:wxy 校對:wxy
本文轉載來自 Linux 中國: https://github.com/Linux-CN/archive